Approach 1: Using the datetimepicker plugin available in jQuery.
- There are two input elements each with a class of datetimepicker.
- We select these elements using the jQuery class selector [$(“class-name”)].
- Apply the datetimepicker() method from the plugin on each input element using the each() method in jQuery.
- The this object in jQuery is used to refer to these input elements such that the datetimepicker() method can be applied to each of them.
CDN Links:
<link rel=”stylesheet” href=”https://cdnjs.cloudflare.com/ajax/libs/jquery-datetimepicker/2.5.20/jquery.datetimepicker.min.css” />
<script src=”https://cdnjs.cloudflare.com/ajax/libs/jquery-datetimepicker/2.5.20/jquery.datetimepicker.full.min.js”></script>
Note: These CDN links must be included in the head tag in order to utilize all functionalities of the datetimepicker plugin.
Example: The following code demonstrates the date picker feature of the plugin.
<!DOCTYPE html>
<html>
<head>
<!-- jQuery CDN -->
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js">
</script>
<!-- CSS CDN -->
<link rel="stylesheet"
href=
"https://cdnjs.cloudflare.com/ajax/libs/jquery-datetimepicker/2.5.20/jquery.datetimepicker.min.css"
/>
<!-- datetimepicker jQuery CDN -->
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/jquery-datetimepicker/2.5.20/jquery.datetimepicker.full.min.js">
</script>
<!-- Basic inline styling -->
<style>
body {
text-align: center;
}
p {
font-size: 25px;
font-weight: bold;
}
</style>
</head>
<body>
<h1 style="color:green">GeeksforGeeks</h1>
<p>jQuery - Set datetimepicker on textbox click</p>
<input type="text"
class="datetimepicker" />
<input type="text"
class="datetimepicker" />
<script type="text/javascript">
$(".datetimepicker").each(function () {
$(this).datetimepicker();
});
</script>
</body>
</html>
Output:
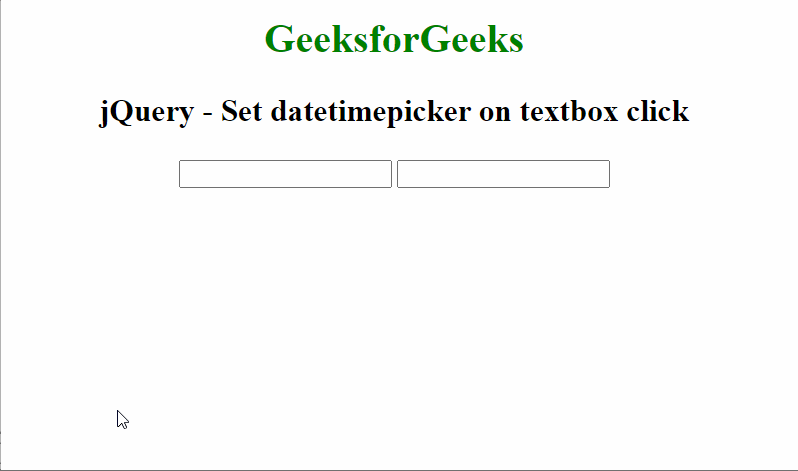